How to take your Python Game from Zero To Hero
Well Python today is a tool which is in everybody's Arsenal, So why not take this to next level. Follow these tips for improving your python game.
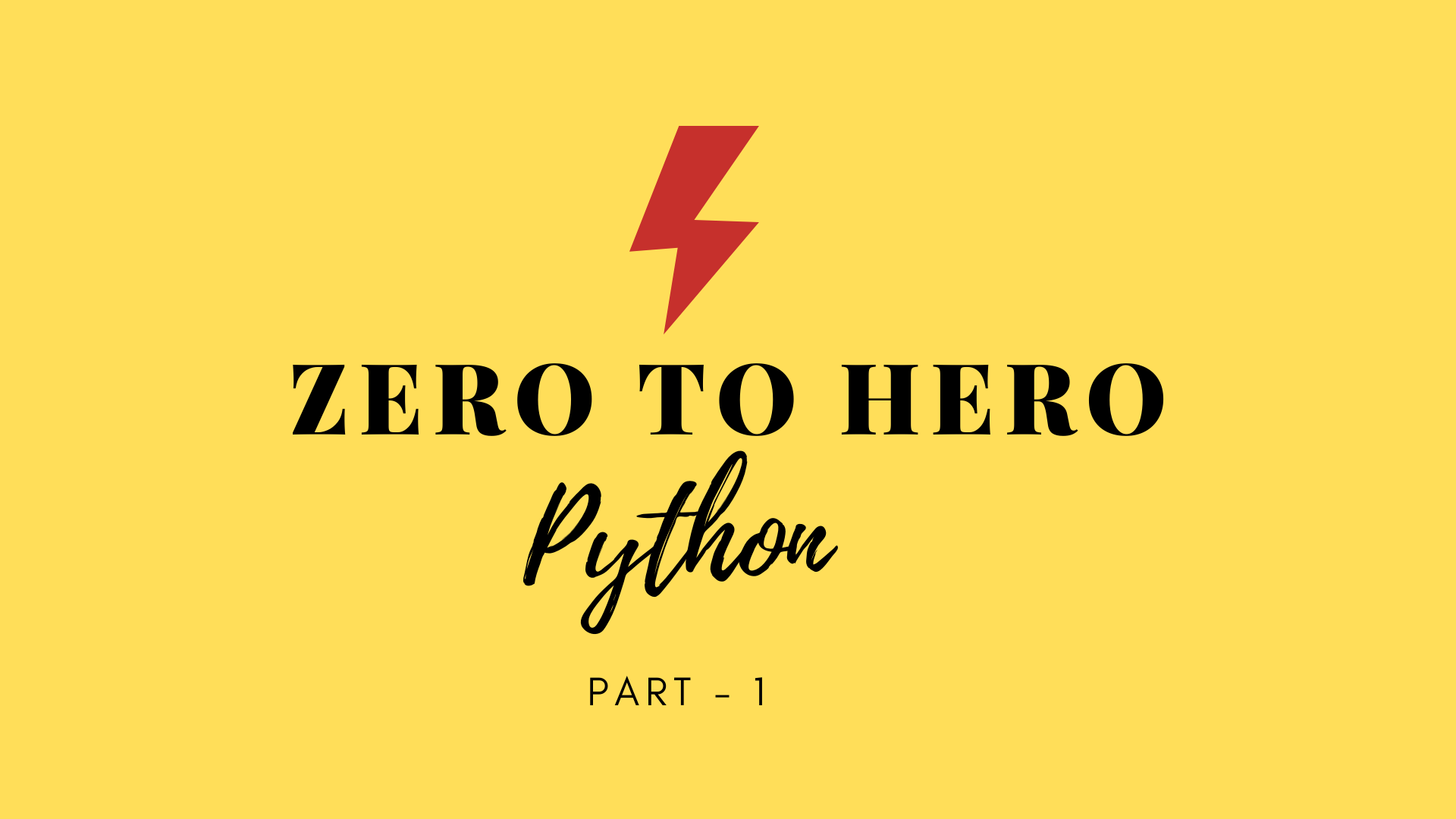
Tips and Tricks For More Attractive & Efficient Python Code
Well Python can be considered as Jack of Trades, And tweaking some parts not only make it look good but also make it more efficient and human readable.
1. Use Variable with if statement.
Sometimes people compare the True
& False
in if statement, which is okay but if its used directly for the condition, It becomes more readable and also serves the condition perfectly.
# 1. Use Variable with if statement.
allow = True
if allow == True:
print("This is allowed")
else:
print("This is not allowed")
#Instead use
allow = True
if allow:
print("This is allowed")
else:
print("This is not allowed")
2. Update index variable using enumerate
Okay so how many times you have used an index variable in iterators. Well we all required it, at someplace but the better way is to use a enumerator.
# 2. Using Enums for index vars
names = ['Peter', 'Karen', 'Parker', 'Sofia']
for index, name in enumerator(names, 1):
print("{}. {}".format(index, name))
#Output
'''
1. Peter
2. Karen
3. Parker
4. Sofia
'''
3. For ~ Else
Well this I have read on the blog on Dev.to and found worth sharing it. So many times we have to check some condition in an iterator and an else condition if we doesn’t get a match.
The Best solution can be paring for with else
# 3. Using For ~ Else
names = ['Peter', 'Karen', 'Parker', 'Sofia']
for name in names:
if name == 'Parker':
print('Name Found')
break
else:
print("Can't find name")
4. Using String Formatting
So it is a been there done that kind of situation. I used to add strings using
+
, but then when I started using String formatting, there was no going back.
# 4. String formatting
# Should not do
print("Hello"+" World"+" This"+" is"+" Python")
# Insted use
print("{} {} {} {} {}".format("Hello","World","This","is","Python")
# If in Python 3.8
name = "Peter"
age = 10
print(f"{name=} {age=}")
#Output: 'name=Peter age=10'
5. Using map and split for taking multiple inputs
This is more important for those trying Competitive Coding using Python,
and there the general input format is inputs sperated by spaces
# 5. Taking space seperated numbers as inputs
# Input: 10 12 13
a, b, c = map(int, input().split())
print(f"{a=} {b=} {c=}")
#Output: 'a=10 b=12 c=13'
6. Using Lambda and Sorted to sort dictionary
Well dictionary are by default constructed random but sometimes we need to sort it, on the basis of key, or maybe on the basis of value.
So what can be done is us a sorted
function with key of lambda
# 6. Sorting Dictionary
dictionary = {'key1':1, 'key2':2, 'key3':3}
# Sorting by the value
sorted(dictionary.items(), key= lambda x:x[1])
# Output [('key1', 1), ('key2', 2), ('key3', 3)]
# Sorting by the key
sorted(dictionary.items(), key= lambda x:x[0])
7. Swapping 2 numbers
When using languages like C
and C++
, there is a requirement of 3 variables for swapping a numbers but in python it can be done pretty easily.
# 7. Swap Two numbers
num1 = 10
num2 = 20
print(f"{num1=}, {num2=}")
#Output: 'num1=10, num2=20'
num1, num2 = num2, num1
print(f"{num1=}, {num2=}")
#Output: 'num1=20, num2=10'
8. Checking Existence of a key in a Dictionary
Sometimes we need to see if a key exist in the dictionary, Now one of the ways is to get the list of keys and then check. Other is mentioned here
# 8. To Match Keys in a dictionary
data = {"key1":"value1", "key2":"value2", "key3":"value3"}
# Matching keys
for "key2" in data:
print("Key2 Exist")
Reviews
If You find it interesting!! we would really like to hear from you.
Ping us at Instagram/@the.blur.code
If you want articles on Any topics dm us on insta.
Thanks for reading!!
Happy Coding